mirror of
https://github.com/DocNR/POWR.git
synced 2025-04-19 10:51:19 +00:00
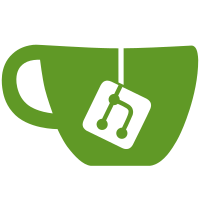
- Update outdated Expo packages to latest compatible versions - Remove unmaintained expo-random package - Remove unnecessary @types/react-native package - Configure eas.json with preview and production profiles for iOS - Fix updates URL in app.json with correct project ID - Add /android and /ios to .gitignore to resolve workflow conflict - Create comprehensive iOS TestFlight submission guide - Add production flag in theme constants - Hide development-only Programs tab in production builds - Remove debug UI and console logs from social feed in production - Update CHANGELOG.md with TestFlight preparation changes All checks from expo-doctor now pass (15/15).
68 lines
2.1 KiB
TypeScript
68 lines
2.1 KiB
TypeScript
// app/(tabs)/library/_layout.tsx
|
|
import { View } from 'react-native';
|
|
import { createMaterialTopTabNavigator } from '@react-navigation/material-top-tabs';
|
|
import ExercisesScreen from './exercises';
|
|
import TemplatesScreen from './templates';
|
|
import ProgramsScreen from './programs';
|
|
import Header from '@/components/Header';
|
|
import { useTheme } from '@react-navigation/native';
|
|
import type { CustomTheme } from '@/lib/theme';
|
|
import { TabScreen } from '@/components/layout/TabScreen';
|
|
import { IS_PRODUCTION } from '@/lib/theme/constants';
|
|
|
|
const Tab = createMaterialTopTabNavigator();
|
|
|
|
export default function LibraryLayout() {
|
|
const theme = useTheme() as CustomTheme;
|
|
|
|
return (
|
|
<TabScreen>
|
|
<Header useLogo={true} showNotifications={true} />
|
|
|
|
<Tab.Navigator
|
|
initialRouteName="templates"
|
|
screenOptions={{
|
|
tabBarActiveTintColor: theme.colors.tabIndicator,
|
|
tabBarInactiveTintColor: theme.colors.tabInactive,
|
|
tabBarLabelStyle: {
|
|
fontSize: 14,
|
|
textTransform: 'capitalize',
|
|
fontWeight: 'bold',
|
|
},
|
|
tabBarIndicatorStyle: {
|
|
backgroundColor: theme.colors.tabIndicator,
|
|
height: 2,
|
|
},
|
|
tabBarStyle: {
|
|
backgroundColor: theme.colors.background,
|
|
elevation: 0,
|
|
shadowOpacity: 0,
|
|
borderBottomWidth: 1,
|
|
borderBottomColor: theme.colors.border,
|
|
},
|
|
tabBarPressColor: theme.colors.primary,
|
|
}}
|
|
>
|
|
<Tab.Screen
|
|
name="exercises"
|
|
component={ExercisesScreen}
|
|
options={{ title: 'Exercises' }}
|
|
/>
|
|
<Tab.Screen
|
|
name="templates"
|
|
component={TemplatesScreen}
|
|
options={{ title: 'Templates' }}
|
|
/>
|
|
{/* Only show Programs tab in development builds */}
|
|
{!IS_PRODUCTION && (
|
|
<Tab.Screen
|
|
name="programs"
|
|
component={ProgramsScreen}
|
|
options={{ title: 'Programs' }}
|
|
/>
|
|
)}
|
|
</Tab.Navigator>
|
|
</TabScreen>
|
|
);
|
|
}
|