mirror of
https://github.com/Stirling-Tools/Stirling-PDF.git
synced 2025-06-23 16:05:09 +00:00
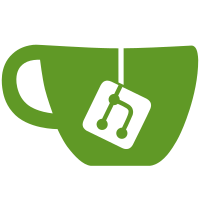
# Description of Changes Please provide a summary of the changes, including: - What was changed - Why the change was made - Any challenges encountered Closes #(issue_number) --- ## Checklist ### General - [ ] I have read the [Contribution Guidelines](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/CONTRIBUTING.md) - [ ] I have read the [Stirling-PDF Developer Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md) (if applicable) - [ ] I have read the [How to add new languages to Stirling-PDF](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md) (if applicable) - [ ] I have performed a self-review of my own code - [ ] My changes generate no new warnings ### Documentation - [ ] I have updated relevant docs on [Stirling-PDF's doc repo](https://github.com/Stirling-Tools/Stirling-Tools.github.io/blob/main/docs/) (if functionality has heavily changed) - [ ] I have read the section [Add New Translation Tags](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md#add-new-translation-tags) (for new translation tags only) ### UI Changes (if applicable) - [ ] Screenshots or videos demonstrating the UI changes are attached (e.g., as comments or direct attachments in the PR) ### Testing (if applicable) - [ ] I have tested my changes locally. Refer to the [Testing Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md#6-testing) for more details.
57 lines
2.1 KiB
Java
57 lines
2.1 KiB
Java
package stirling.software.SPDF.service;
|
|
|
|
import java.io.IOException;
|
|
import java.util.Arrays;
|
|
import java.util.HashSet;
|
|
import java.util.Set;
|
|
import java.util.stream.Collectors;
|
|
|
|
import org.springframework.core.io.Resource;
|
|
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
|
|
import org.springframework.stereotype.Service;
|
|
|
|
import lombok.extern.slf4j.Slf4j;
|
|
import stirling.software.SPDF.model.ApplicationProperties;
|
|
|
|
@Service
|
|
@Slf4j
|
|
public class LanguageService {
|
|
|
|
private final ApplicationProperties applicationProperties;
|
|
private final PathMatchingResourcePatternResolver resourcePatternResolver =
|
|
new PathMatchingResourcePatternResolver();
|
|
|
|
public LanguageService(ApplicationProperties applicationProperties) {
|
|
this.applicationProperties = applicationProperties;
|
|
}
|
|
|
|
public Set<String> getSupportedLanguages() {
|
|
try {
|
|
Resource[] resources =
|
|
resourcePatternResolver.getResources("classpath*:messages_*.properties");
|
|
|
|
return Arrays.stream(resources)
|
|
.map(Resource::getFilename)
|
|
.filter(
|
|
filename ->
|
|
filename != null
|
|
&& filename.startsWith("messages_")
|
|
&& filename.endsWith(".properties"))
|
|
.map(filename -> filename.replace("messages_", "").replace(".properties", ""))
|
|
.filter(
|
|
languageCode -> {
|
|
Set<String> allowedLanguages =
|
|
new HashSet<>(applicationProperties.getUi().getLanguages());
|
|
return allowedLanguages.isEmpty()
|
|
|| allowedLanguages.contains(languageCode)
|
|
|| "en_GB".equals(languageCode);
|
|
})
|
|
.collect(Collectors.toSet());
|
|
|
|
} catch (IOException e) {
|
|
log.error("Error retrieving supported languages", e);
|
|
return new HashSet<>();
|
|
}
|
|
}
|
|
}
|