mirror of
https://github.com/Stirling-Tools/Stirling-PDF.git
synced 2025-05-15 18:55:56 +00:00
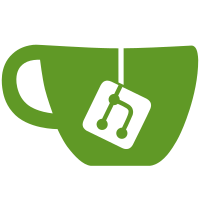
# Description of Changes This pull request includes several changes primarily focused on improving configuration management, removing deprecated methods, and updating paths for external dependencies. The most important changes are summarized below: ### Configuration Management Improvements: * Added a new `RuntimePathConfig` class to manage dynamic paths for operations and pipeline configurations (`src/main/java/stirling/software/SPDF/config/RuntimePathConfig.java`). * Removed the `bookAndHtmlFormatsInstalled` bean and its associated logic from `AppConfig` and `EndpointConfiguration` (`src/main/java/stirling/software/SPDF/config/AppConfig.java`, `src/main/java/stirling/software/SPDF/config/EndpointConfiguration.java`). [[1]](diffhunk://#diff-4d774ec79aa55750c0a4739bee971b68877078b73654e863fd40ee924347e143L130-L138) [[2]](diffhunk://#diff-750f31f6ecbd64b025567108a33775cad339e835a04360affff82a09410b697dL12-L35) [[3]](diffhunk://#diff-750f31f6ecbd64b025567108a33775cad339e835a04360affff82a09410b697dL275-L280) ### External Dependency Path Updates: * Updated paths for `weasyprint` and `unoconvert` in `ExternalAppDepConfig` to use values from `RuntimePathConfig` (`src/main/java/stirling/software/SPDF/config/ExternalAppDepConfig.java`). [[1]](diffhunk://#diff-c47af298c07c2622aa98b038b78822c56bdb002de71081e102d344794e7832a6R12-L33) [[2]](diffhunk://#diff-c47af298c07c2622aa98b038b78822c56bdb002de71081e102d344794e7832a6L104-R115) ### Minor Adjustments: * Corrected a typo from "Unoconv" to "Unoconvert" in `EndpointConfiguration` (`src/main/java/stirling/software/SPDF/config/EndpointConfiguration.java`). --- ## Checklist ### General - [ ] I have read the [Contribution Guidelines](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/CONTRIBUTING.md) - [ ] I have read the [Stirling-PDF Developer Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md) (if applicable) - [ ] I have read the [How to add new languages to Stirling-PDF](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md) (if applicable) - [ ] I have performed a self-review of my own code - [ ] My changes generate no new warnings ### Documentation - [ ] I have updated relevant docs on [Stirling-PDF's doc repo](https://github.com/Stirling-Tools/Stirling-Tools.github.io/blob/main/docs/) (if functionality has heavily changed) - [ ] I have read the section [Add New Translation Tags](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md#add-new-translation-tags) (for new translation tags only) ### UI Changes (if applicable) - [ ] Screenshots or videos demonstrating the UI changes are attached (e.g., as comments or direct attachments in the PR) ### Testing (if applicable) - [ ] I have tested my changes locally. Refer to the [Testing Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md#6-testing) for more details.
341 lines
13 KiB
Java
341 lines
13 KiB
Java
package stirling.software.SPDF.controller.web;
|
|
|
|
import java.time.Duration;
|
|
import java.time.LocalDateTime;
|
|
import java.util.*;
|
|
import java.util.stream.Collectors;
|
|
|
|
import org.springframework.http.HttpStatus;
|
|
import org.springframework.http.ResponseEntity;
|
|
import org.springframework.web.bind.annotation.GetMapping;
|
|
import org.springframework.web.bind.annotation.RequestMapping;
|
|
import org.springframework.web.bind.annotation.RequestParam;
|
|
import org.springframework.web.bind.annotation.RestController;
|
|
|
|
import io.micrometer.core.instrument.Counter;
|
|
import io.micrometer.core.instrument.MeterRegistry;
|
|
import io.swagger.v3.oas.annotations.Operation;
|
|
import io.swagger.v3.oas.annotations.Parameter;
|
|
import io.swagger.v3.oas.annotations.tags.Tag;
|
|
|
|
import jakarta.annotation.PostConstruct;
|
|
|
|
import lombok.extern.slf4j.Slf4j;
|
|
|
|
import stirling.software.SPDF.config.StartupApplicationListener;
|
|
import stirling.software.SPDF.model.ApplicationProperties;
|
|
|
|
@RestController
|
|
@RequestMapping("/api/v1/info")
|
|
@Tag(name = "Info", description = "Info APIs")
|
|
@Slf4j
|
|
public class MetricsController {
|
|
|
|
private final ApplicationProperties applicationProperties;
|
|
|
|
private final MeterRegistry meterRegistry;
|
|
|
|
private boolean metricsEnabled;
|
|
|
|
public MetricsController(
|
|
ApplicationProperties applicationProperties, MeterRegistry meterRegistry) {
|
|
this.applicationProperties = applicationProperties;
|
|
this.meterRegistry = meterRegistry;
|
|
}
|
|
|
|
@PostConstruct
|
|
public void init() {
|
|
Boolean metricsEnabled = applicationProperties.getMetrics().getEnabled();
|
|
if (metricsEnabled == null) metricsEnabled = true;
|
|
this.metricsEnabled = metricsEnabled;
|
|
}
|
|
|
|
@GetMapping("/status")
|
|
@Operation(
|
|
summary = "Application status and version",
|
|
description =
|
|
"This endpoint returns the status of the application and its version number.")
|
|
public ResponseEntity<?> getStatus() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
Map<String, String> status = new HashMap<>();
|
|
status.put("status", "UP");
|
|
status.put("version", getClass().getPackage().getImplementationVersion());
|
|
return ResponseEntity.ok(status);
|
|
}
|
|
|
|
@GetMapping("/load")
|
|
@Operation(
|
|
summary = "GET request count",
|
|
description =
|
|
"This endpoint returns the total count of GET requests for a specific endpoint or all endpoints.")
|
|
public ResponseEntity<?> getPageLoads(
|
|
@RequestParam(required = false, name = "endpoint") @Parameter(description = "endpoint")
|
|
Optional<String> endpoint) {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
double count = getRequestCount("GET", endpoint);
|
|
return ResponseEntity.ok(count);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
@GetMapping("/load/unique")
|
|
@Operation(
|
|
summary = "Unique users count for GET requests",
|
|
description =
|
|
"This endpoint returns the count of unique users for GET requests for a specific endpoint or all endpoints.")
|
|
public ResponseEntity<?> getUniquePageLoads(
|
|
@RequestParam(required = false, name = "endpoint") @Parameter(description = "endpoint")
|
|
Optional<String> endpoint) {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
double count = getUniqueUserCount("GET", endpoint);
|
|
return ResponseEntity.ok(count);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
@GetMapping("/load/all")
|
|
@Operation(
|
|
summary = "GET requests count for all endpoints",
|
|
description = "This endpoint returns the count of GET requests for each endpoint.")
|
|
public ResponseEntity<?> getAllEndpointLoads() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
List<EndpointCount> results = getEndpointCounts("GET");
|
|
return ResponseEntity.ok(results);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
@GetMapping("/load/all/unique")
|
|
@Operation(
|
|
summary = "Unique users count for GET requests for all endpoints",
|
|
description =
|
|
"This endpoint returns the count of unique users for GET requests for each endpoint.")
|
|
public ResponseEntity<?> getAllUniqueEndpointLoads() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
List<EndpointCount> results = getUniqueUserCounts("GET");
|
|
return ResponseEntity.ok(results);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
@GetMapping("/requests")
|
|
@Operation(
|
|
summary = "POST request count",
|
|
description =
|
|
"This endpoint returns the total count of POST requests for a specific endpoint or all endpoints.")
|
|
public ResponseEntity<?> getTotalRequests(
|
|
@RequestParam(required = false, name = "endpoint") @Parameter(description = "endpoint")
|
|
Optional<String> endpoint) {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
double count = getRequestCount("POST", endpoint);
|
|
return ResponseEntity.ok(count);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.ok(-1);
|
|
}
|
|
}
|
|
|
|
@GetMapping("/requests/unique")
|
|
@Operation(
|
|
summary = "Unique users count for POST requests",
|
|
description =
|
|
"This endpoint returns the count of unique users for POST requests for a specific endpoint or all endpoints.")
|
|
public ResponseEntity<?> getUniqueTotalRequests(
|
|
@RequestParam(required = false, name = "endpoint") @Parameter(description = "endpoint")
|
|
Optional<String> endpoint) {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
double count = getUniqueUserCount("POST", endpoint);
|
|
return ResponseEntity.ok(count);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.ok(-1);
|
|
}
|
|
}
|
|
|
|
@GetMapping("/requests/all")
|
|
@Operation(
|
|
summary = "POST requests count for all endpoints",
|
|
description = "This endpoint returns the count of POST requests for each endpoint.")
|
|
public ResponseEntity<?> getAllPostRequests() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
List<EndpointCount> results = getEndpointCounts("POST");
|
|
return ResponseEntity.ok(results);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
@GetMapping("/requests/all/unique")
|
|
@Operation(
|
|
summary = "Unique users count for POST requests for all endpoints",
|
|
description =
|
|
"This endpoint returns the count of unique users for POST requests for each endpoint.")
|
|
public ResponseEntity<?> getAllUniquePostRequests() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
try {
|
|
List<EndpointCount> results = getUniqueUserCounts("POST");
|
|
return ResponseEntity.ok(results);
|
|
} catch (Exception e) {
|
|
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
|
|
}
|
|
}
|
|
|
|
private double getRequestCount(String method, Optional<String> endpoint) {
|
|
log.info(
|
|
"Getting request count for method: {}, endpoint: {}",
|
|
method,
|
|
endpoint.orElse("all"));
|
|
double count =
|
|
meterRegistry.find("http.requests").tag("method", method).counters().stream()
|
|
.filter(
|
|
counter ->
|
|
!endpoint.isPresent()
|
|
|| endpoint.get()
|
|
.equals(counter.getId().getTag("uri")))
|
|
.mapToDouble(Counter::count)
|
|
.sum();
|
|
log.info("Request count: {}", count);
|
|
return count;
|
|
}
|
|
|
|
private List<EndpointCount> getEndpointCounts(String method) {
|
|
log.info("Getting endpoint counts for method: {}", method);
|
|
Map<String, Double> counts = new HashMap<>();
|
|
meterRegistry
|
|
.find("http.requests")
|
|
.tag("method", method)
|
|
.counters()
|
|
.forEach(
|
|
counter -> {
|
|
String uri = counter.getId().getTag("uri");
|
|
counts.merge(uri, counter.count(), Double::sum);
|
|
});
|
|
List<EndpointCount> result =
|
|
counts.entrySet().stream()
|
|
.map(entry -> new EndpointCount(entry.getKey(), entry.getValue()))
|
|
.sorted(Comparator.comparing(EndpointCount::getCount).reversed())
|
|
.collect(Collectors.toList());
|
|
log.info("Found {} endpoints with counts", result.size());
|
|
return result;
|
|
}
|
|
|
|
private double getUniqueUserCount(String method, Optional<String> endpoint) {
|
|
log.info(
|
|
"Getting unique user count for method: {}, endpoint: {}",
|
|
method,
|
|
endpoint.orElse("all"));
|
|
Set<String> uniqueUsers = new HashSet<>();
|
|
meterRegistry.find("http.requests").tag("method", method).counters().stream()
|
|
.filter(
|
|
counter ->
|
|
!endpoint.isPresent()
|
|
|| endpoint.get().equals(counter.getId().getTag("uri")))
|
|
.forEach(
|
|
counter -> {
|
|
String session = counter.getId().getTag("session");
|
|
if (session != null) {
|
|
uniqueUsers.add(session);
|
|
}
|
|
});
|
|
log.info("Unique user count: {}", uniqueUsers.size());
|
|
return uniqueUsers.size();
|
|
}
|
|
|
|
private List<EndpointCount> getUniqueUserCounts(String method) {
|
|
log.info("Getting unique user counts for method: {}", method);
|
|
Map<String, Set<String>> uniqueUsers = new HashMap<>();
|
|
meterRegistry
|
|
.find("http.requests")
|
|
.tag("method", method)
|
|
.counters()
|
|
.forEach(
|
|
counter -> {
|
|
String uri = counter.getId().getTag("uri");
|
|
String session = counter.getId().getTag("session");
|
|
if (uri != null && session != null) {
|
|
uniqueUsers.computeIfAbsent(uri, k -> new HashSet<>()).add(session);
|
|
}
|
|
});
|
|
List<EndpointCount> result =
|
|
uniqueUsers.entrySet().stream()
|
|
.map(entry -> new EndpointCount(entry.getKey(), entry.getValue().size()))
|
|
.sorted(Comparator.comparing(EndpointCount::getCount).reversed())
|
|
.collect(Collectors.toList());
|
|
log.info("Found {} endpoints with unique user counts", result.size());
|
|
return result;
|
|
}
|
|
|
|
@GetMapping("/uptime")
|
|
public ResponseEntity<?> getUptime() {
|
|
if (!metricsEnabled) {
|
|
return ResponseEntity.status(HttpStatus.FORBIDDEN).body("This endpoint is disabled.");
|
|
}
|
|
LocalDateTime now = LocalDateTime.now();
|
|
Duration uptime = Duration.between(StartupApplicationListener.startTime, now);
|
|
return ResponseEntity.ok(formatDuration(uptime));
|
|
}
|
|
|
|
private String formatDuration(Duration duration) {
|
|
long days = duration.toDays();
|
|
long hours = duration.toHoursPart();
|
|
long minutes = duration.toMinutesPart();
|
|
long seconds = duration.toSecondsPart();
|
|
return String.format("%dd %dh %dm %ds", days, hours, minutes, seconds);
|
|
}
|
|
|
|
public static class EndpointCount {
|
|
|
|
private String endpoint;
|
|
|
|
private double count;
|
|
|
|
public EndpointCount(String endpoint, double count) {
|
|
this.endpoint = endpoint;
|
|
this.count = count;
|
|
}
|
|
|
|
public String getEndpoint() {
|
|
return endpoint;
|
|
}
|
|
|
|
public void setEndpoint(String endpoint) {
|
|
this.endpoint = endpoint;
|
|
}
|
|
|
|
public double getCount() {
|
|
return count;
|
|
}
|
|
|
|
public void setCount(double count) {
|
|
this.count = count;
|
|
}
|
|
}
|
|
}
|