mirror of
https://github.com/Stirling-Tools/Stirling-PDF.git
synced 2025-05-15 10:45:54 +00:00
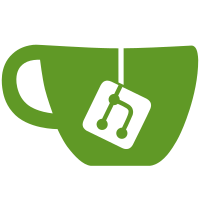
# Description This pull request includes several changes aimed at improving the code structure and removing redundant code. The most significant changes involve reordering methods, removing unnecessary annotations, and refactoring constructors to use dependency injection. Autowired now comes via constructor (which also doesn't need autowired annotation as its done by default for configuration) ## Checklist - [ ] I have read the [Contribution Guidelines](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/CONTRIBUTING.md) - [ ] I have performed a self-review of my own code - [ ] I have attached images of the change if it is UI based - [ ] I have commented my code, particularly in hard-to-understand areas - [ ] If my code has heavily changed functionality I have updated relevant docs on [Stirling-PDFs doc repo](https://github.com/Stirling-Tools/Stirling-Tools.github.io/blob/main/docs/) - [ ] My changes generate no new warnings - [ ] I have read the section [Add New Translation Tags](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md#add-new-translation-tags) (for new translation tags only)
148 lines
3.5 KiB
Java
148 lines
3.5 KiB
Java
package stirling.software.SPDF.model;
|
|
|
|
import java.io.Serializable;
|
|
import java.util.HashMap;
|
|
import java.util.HashSet;
|
|
import java.util.Map;
|
|
import java.util.Set;
|
|
import java.util.stream.Collectors;
|
|
|
|
import jakarta.persistence.*;
|
|
|
|
@Entity
|
|
@Table(name = "users")
|
|
public class User implements Serializable {
|
|
|
|
private static final long serialVersionUID = 1L;
|
|
|
|
@Id
|
|
@GeneratedValue(strategy = GenerationType.IDENTITY)
|
|
@Column(name = "user_id")
|
|
private Long id;
|
|
|
|
@Column(name = "username", unique = true)
|
|
private String username;
|
|
|
|
@Column(name = "password")
|
|
private String password;
|
|
|
|
@Column(name = "apiKey")
|
|
private String apiKey;
|
|
|
|
@Column(name = "enabled")
|
|
private boolean enabled;
|
|
|
|
@Column(name = "isFirstLogin")
|
|
private Boolean isFirstLogin = false;
|
|
|
|
@Column(name = "roleName")
|
|
private String roleName;
|
|
|
|
@Column(name = "authenticationtype")
|
|
private String authenticationType;
|
|
|
|
@OneToMany(fetch = FetchType.EAGER, cascade = CascadeType.ALL, mappedBy = "user")
|
|
private Set<Authority> authorities = new HashSet<>();
|
|
|
|
@ElementCollection
|
|
@MapKeyColumn(name = "setting_key")
|
|
@Lob
|
|
@Column(name = "setting_value", columnDefinition = "CLOB")
|
|
@CollectionTable(name = "user_settings", joinColumns = @JoinColumn(name = "user_id"))
|
|
private Map<String, String> settings = new HashMap<>(); // Key-value pairs of settings.
|
|
|
|
public String getRoleName() {
|
|
return Role.getRoleNameByRoleId(getRolesAsString());
|
|
}
|
|
|
|
public boolean isFirstLogin() {
|
|
return isFirstLogin != null && isFirstLogin;
|
|
}
|
|
|
|
public void setFirstLogin(boolean isFirstLogin) {
|
|
this.isFirstLogin = isFirstLogin;
|
|
}
|
|
|
|
public Long getId() {
|
|
return id;
|
|
}
|
|
|
|
public void setId(Long id) {
|
|
this.id = id;
|
|
}
|
|
|
|
public String getApiKey() {
|
|
return apiKey;
|
|
}
|
|
|
|
public void setApiKey(String apiKey) {
|
|
this.apiKey = apiKey;
|
|
}
|
|
|
|
public Map<String, String> getSettings() {
|
|
return settings;
|
|
}
|
|
|
|
public void setSettings(Map<String, String> settings) {
|
|
this.settings = settings;
|
|
}
|
|
|
|
public String getUsername() {
|
|
return username;
|
|
}
|
|
|
|
public void setUsername(String username) {
|
|
this.username = username;
|
|
}
|
|
|
|
public String getPassword() {
|
|
return password;
|
|
}
|
|
|
|
public void setPassword(String password) {
|
|
this.password = password;
|
|
}
|
|
|
|
public boolean isEnabled() {
|
|
return enabled;
|
|
}
|
|
|
|
public void setEnabled(boolean enabled) {
|
|
this.enabled = enabled;
|
|
}
|
|
|
|
public String getAuthenticationType() {
|
|
return authenticationType;
|
|
}
|
|
|
|
public void setAuthenticationType(AuthenticationType authenticationType) {
|
|
this.authenticationType = authenticationType.toString().toLowerCase();
|
|
}
|
|
|
|
public Set<Authority> getAuthorities() {
|
|
return authorities;
|
|
}
|
|
|
|
public void setAuthorities(Set<Authority> authorities) {
|
|
this.authorities = authorities;
|
|
}
|
|
|
|
public void addAuthorities(Set<Authority> authorities) {
|
|
this.authorities.addAll(authorities);
|
|
}
|
|
|
|
public void addAuthority(Authority authorities) {
|
|
this.authorities.add(authorities);
|
|
}
|
|
|
|
public String getRolesAsString() {
|
|
return this.authorities.stream()
|
|
.map(Authority::getAuthority)
|
|
.collect(Collectors.joining(", "));
|
|
}
|
|
|
|
public boolean hasPassword() {
|
|
return this.password != null && !this.password.isEmpty();
|
|
}
|
|
}
|