mirror of
https://github.com/Stirling-Tools/Stirling-PDF.git
synced 2025-05-14 10:15:55 +00:00
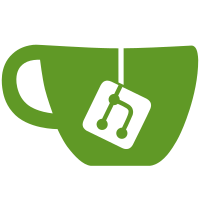
# Description of Changes ### Changes: - Add a new custom select to display fonts correctly and to allow more styling flexibility in Sign PDF feature by hiding the original `<select>` element (`display: none;`) and wrap it with a `<div>` and then create a custom selection menu using `<div>`s and CSS to achieve the required results due to the limitations of `<select>` and `<option>` while still preserving the hidden `<select>` for form submission. ### Why was the change made? 1. A bug that caused font families to not be displayed in Firefox. 2. Select/Option element are not flexible when it comes to styling (compared to DIVs for example) but bullet point `1.` is of higher priority. ### UI Changes: - Dark Mode: - Before:  - After:  - Light Mode: - Before:  - After:  Note: - Changes in `sign.js` are between the lines 95-228, as it seems the file was auto-formatted affecting whitespaces and single_quotes/double_quotes. #### Useful quotes from MDN: > [Styling with CSS](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/option#styling_with_css) Styling the <option> element is highly limited. Options don't inherit the font set on the parent. In Firefox, only [color](https://developer.mozilla.org/en-US/docs/Web/CSS/color) and [background-color](https://developer.mozilla.org/en-US/docs/Web/CSS/background-color) can be set, however in Chrome and Safari it's not possible to set any properties. You can find more details about styling in [our guide to advanced form styling](https://developer.mozilla.org/en-US/docs/Learn_web_development/Extensions/Forms/Advanced_form_styling). #### Useful references: - [Option Element](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/option#styling_with_css) - [Select Element](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select) - [Advanced Form Styling](https://developer.mozilla.org/en-US/docs/Learn_web_development/Extensions/Forms/Advanced_form_styling) Closes #1575 --- ## Checklist ### General - [x] I have read the [Contribution Guidelines](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/CONTRIBUTING.md) - [x] I have read the [Stirling-PDF Developer Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md) (if applicable) - [ ] I have read the [How to add new languages to Stirling-PDF](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md) (if applicable) - [x] I have performed a self-review of my own code - [x] My changes generate no new warnings ### Documentation - [ ] I have updated relevant docs on [Stirling-PDF's doc repo](https://github.com/Stirling-Tools/Stirling-Tools.github.io/blob/main/docs/) (if functionality has heavily changed) - [ ] I have read the section [Add New Translation Tags](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md#add-new-translation-tags) (for new translation tags only) ### UI Changes (if applicable) - [x] Screenshots or videos demonstrating the UI changes are attached (e.g., as comments or direct attachments in the PR) ### Testing (if applicable) - [x] I have tested my changes locally. Refer to the [Testing Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md#6-testing) for more details.
390 lines
12 KiB
JavaScript
390 lines
12 KiB
JavaScript
window.toggleSignatureView = toggleSignatureView;
|
|
window.previewSignature = previewSignature;
|
|
window.addSignatureFromPreview = addSignatureFromPreview;
|
|
window.addDraggableFromPad = addDraggableFromPad;
|
|
window.addDraggableFromText = addDraggableFromText;
|
|
window.goToFirstOrLastPage = goToFirstOrLastPage;
|
|
|
|
let currentPreviewSrc = null;
|
|
|
|
function toggleSignatureView() {
|
|
const gridView = document.getElementById("gridView");
|
|
const listView = document.getElementById("listView");
|
|
const gridText = document.querySelector(".grid-view-text");
|
|
const listText = document.querySelector(".list-view-text");
|
|
|
|
if (gridView.style.display !== "none") {
|
|
gridView.style.display = "none";
|
|
listView.style.display = "block";
|
|
gridText.style.display = "none";
|
|
listText.style.display = "inline";
|
|
} else {
|
|
gridView.style.display = "block";
|
|
listView.style.display = "none";
|
|
gridText.style.display = "inline";
|
|
listText.style.display = "none";
|
|
}
|
|
}
|
|
|
|
function previewSignature(element) {
|
|
const src = element.dataset.src;
|
|
currentPreviewSrc = src;
|
|
|
|
const filename = element.querySelector(".signature-list-name").textContent;
|
|
|
|
const previewImage = document.getElementById("previewImage");
|
|
const previewFileName = document.getElementById("previewFileName");
|
|
|
|
previewImage.src = src;
|
|
previewFileName.textContent = filename;
|
|
|
|
const modal = new bootstrap.Modal(
|
|
document.getElementById("signaturePreview")
|
|
);
|
|
modal.show();
|
|
}
|
|
|
|
function addSignatureFromPreview() {
|
|
if (currentPreviewSrc) {
|
|
DraggableUtils.createDraggableCanvasFromUrl(currentPreviewSrc);
|
|
bootstrap.Modal.getInstance(
|
|
document.getElementById("signaturePreview")
|
|
).hide();
|
|
}
|
|
}
|
|
|
|
let originalFileName = "";
|
|
document
|
|
.querySelector("input[name=pdf-upload]")
|
|
.addEventListener("change", async (event) => {
|
|
const fileInput = event.target;
|
|
fileInput.addEventListener("file-input-change", async (e) => {
|
|
const { allFiles } = e.detail;
|
|
if (allFiles && allFiles.length > 0) {
|
|
const file = allFiles[0];
|
|
originalFileName = file.name.replace(/\.[^/.]+$/, "");
|
|
const pdfData = await file.arrayBuffer();
|
|
pdfjsLib.GlobalWorkerOptions.workerSrc =
|
|
"./pdfjs-legacy/pdf.worker.mjs";
|
|
const pdfDoc = await pdfjsLib.getDocument({ data: pdfData }).promise;
|
|
await DraggableUtils.renderPage(pdfDoc, 0);
|
|
|
|
document.querySelectorAll(".show-on-file-selected").forEach((el) => {
|
|
el.style.cssText = "";
|
|
});
|
|
}
|
|
});
|
|
});
|
|
|
|
document.addEventListener("DOMContentLoaded", () => {
|
|
document.querySelectorAll(".show-on-file-selected").forEach((el) => {
|
|
el.style.cssText = "display:none !important";
|
|
});
|
|
document
|
|
.querySelectorAll(".small-file-container-saved img ")
|
|
.forEach((img) => {
|
|
img.addEventListener("dragstart", (e) => {
|
|
e.dataTransfer.setData("fileUrl", img.src);
|
|
});
|
|
});
|
|
document.addEventListener("keydown", (e) => {
|
|
if (e.key === "Delete") {
|
|
DraggableUtils.deleteDraggableCanvas(DraggableUtils.getLastInteracted());
|
|
}
|
|
});
|
|
|
|
addCustomSelect();
|
|
});
|
|
|
|
function addCustomSelect() {
|
|
let customSelectElementContainer =
|
|
document.getElementById("signFontSelection");
|
|
let originalSelectElement =
|
|
customSelectElementContainer.querySelector("select");
|
|
|
|
let optionsCount = originalSelectElement.length;
|
|
|
|
let selectedItem = createAndStyleSelectedItem();
|
|
|
|
customSelectElementContainer.appendChild(selectedItem);
|
|
|
|
let customSelectionsOptionsContainer = createCustomOptionsContainer();
|
|
createAndAddCustomOptions();
|
|
customSelectElementContainer.appendChild(customSelectionsOptionsContainer);
|
|
|
|
selectedItem.addEventListener("click", function (e) {
|
|
/* When the select box is clicked, close any other select boxes,
|
|
and open/close the current select box: */
|
|
e.stopPropagation();
|
|
closeAllSelect(this);
|
|
this.nextSibling.classList.toggle("select-hide");
|
|
this.classList.toggle("select-arrow-active");
|
|
});
|
|
|
|
function createAndAddCustomOptions() {
|
|
for (let j = 0; j < optionsCount; j++) {
|
|
/* For each option in the original select element,
|
|
create a new DIV that will act as an option item: */
|
|
let customOptionItem = createAndStyleCustomOption(j);
|
|
|
|
customOptionItem.addEventListener("click", onCustomOptionClick);
|
|
customSelectionsOptionsContainer.appendChild(customOptionItem);
|
|
}
|
|
}
|
|
|
|
function createCustomOptionsContainer() {
|
|
let customSelectionsOptionsContainer = document.createElement("DIV");
|
|
customSelectionsOptionsContainer.setAttribute(
|
|
"class",
|
|
"select-items select-hide"
|
|
);
|
|
return customSelectionsOptionsContainer;
|
|
}
|
|
|
|
function createAndStyleSelectedItem() {
|
|
let selectedItem = document.createElement("DIV");
|
|
selectedItem.setAttribute("class", "select-selected");
|
|
selectedItem.innerHTML =
|
|
originalSelectElement.options[
|
|
originalSelectElement.selectedIndex
|
|
].innerHTML;
|
|
|
|
selectedItem.style.fontFamily = window.getComputedStyle(
|
|
originalSelectElement.options[originalSelectElement.selectedIndex]
|
|
).fontFamily;
|
|
return selectedItem;
|
|
}
|
|
|
|
function onCustomOptionClick(e) {
|
|
/* When an item is clicked, update the original select box,
|
|
and the selected item: */
|
|
let selectElement =
|
|
this.parentNode.parentNode.getElementsByTagName("select")[0];
|
|
let optionsCount = selectElement.length;
|
|
let currentlySelectedCustomOption = this.parentNode.previousSibling;
|
|
for (let i = 0; i < optionsCount; i++) {
|
|
if (selectElement.options[i].innerHTML == this.innerHTML) {
|
|
selectElement.selectedIndex = i;
|
|
currentlySelectedCustomOption.innerHTML = this.innerHTML;
|
|
currentlySelectedCustomOption.style.fontFamily = this.style.fontFamily;
|
|
|
|
let previouslySelectedOption =
|
|
this.parentNode.getElementsByClassName("same-as-selected");
|
|
|
|
if (previouslySelectedOption && previouslySelectedOption.length > 0)
|
|
previouslySelectedOption[0].classList.remove("same-as-selected");
|
|
|
|
this.classList.add("same-as-selected");
|
|
break;
|
|
}
|
|
}
|
|
currentlySelectedCustomOption.click();
|
|
}
|
|
|
|
function createAndStyleCustomOption(j) {
|
|
let customOptionItem = document.createElement("DIV");
|
|
customOptionItem.innerHTML = originalSelectElement.options[j].innerHTML;
|
|
customOptionItem.classList.add(originalSelectElement.options[j].className);
|
|
customOptionItem.style.fontFamily = window.getComputedStyle(
|
|
originalSelectElement.options[j]
|
|
).fontFamily;
|
|
|
|
if (j == originalSelectElement.selectedIndex)
|
|
customOptionItem.classList.add("same-as-selected");
|
|
return customOptionItem;
|
|
}
|
|
|
|
function closeAllSelect(element) {
|
|
/* A function that will close all select boxes in the document,
|
|
except the current select box: */
|
|
let allSelectedOptions = document.getElementsByClassName("select-selected");
|
|
let allSelectedOptionsCount = allSelectedOptions.length;
|
|
let indicesOfContainersToHide = [];
|
|
for (let i = 0; i < allSelectedOptionsCount; i++) {
|
|
if (element == allSelectedOptions[i]) {
|
|
indicesOfContainersToHide.push(i);
|
|
} else {
|
|
allSelectedOptions[i].classList.remove("select-arrow-active");
|
|
}
|
|
}
|
|
|
|
hideOptionsContainers(indicesOfContainersToHide);
|
|
}
|
|
|
|
/* If the user clicks anywhere outside the select box,
|
|
then close all select boxes: */
|
|
document.addEventListener("click", closeAllSelect);
|
|
|
|
function hideOptionsContainers(containersIndices) {
|
|
let allOptionsContainers = document.getElementsByClassName("select-items");
|
|
let allSelectionListsContainerCount = allOptionsContainers.length;
|
|
for (let i = 0; i < allSelectionListsContainerCount; i++) {
|
|
if (containersIndices.indexOf(i)) {
|
|
allOptionsContainers[i].classList.add("select-hide");
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
const imageUpload = document.querySelector("input[name=image-upload]");
|
|
imageUpload.addEventListener("change", (e) => {
|
|
if (!e.target.files) return;
|
|
for (const imageFile of e.target.files) {
|
|
var reader = new FileReader();
|
|
reader.readAsDataURL(imageFile);
|
|
reader.onloadend = function (e) {
|
|
DraggableUtils.createDraggableCanvasFromUrl(e.target.result);
|
|
};
|
|
}
|
|
});
|
|
|
|
const signaturePadCanvas = document.getElementById("drawing-pad-canvas");
|
|
const signaturePad = new SignaturePad(signaturePadCanvas, {
|
|
minWidth: 1,
|
|
maxWidth: 2,
|
|
penColor: "black",
|
|
});
|
|
|
|
function addDraggableFromPad() {
|
|
if (signaturePad.isEmpty()) return;
|
|
const startTime = Date.now();
|
|
const croppedDataUrl = getCroppedCanvasDataUrl(signaturePadCanvas);
|
|
console.log(Date.now() - startTime);
|
|
DraggableUtils.createDraggableCanvasFromUrl(croppedDataUrl);
|
|
}
|
|
|
|
function getCroppedCanvasDataUrl(canvas) {
|
|
let originalCtx = canvas.getContext("2d");
|
|
let originalWidth = canvas.width;
|
|
let originalHeight = canvas.height;
|
|
let imageData = originalCtx.getImageData(0, 0, originalWidth, originalHeight);
|
|
|
|
let minX = originalWidth + 1,
|
|
maxX = -1,
|
|
minY = originalHeight + 1,
|
|
maxY = -1,
|
|
x = 0,
|
|
y = 0,
|
|
currentPixelColorValueIndex;
|
|
|
|
for (y = 0; y < originalHeight; y++) {
|
|
for (x = 0; x < originalWidth; x++) {
|
|
currentPixelColorValueIndex = (y * originalWidth + x) * 4;
|
|
let currentPixelAlphaValue =
|
|
imageData.data[currentPixelColorValueIndex + 3];
|
|
if (currentPixelAlphaValue > 0) {
|
|
if (minX > x) minX = x;
|
|
if (maxX < x) maxX = x;
|
|
if (minY > y) minY = y;
|
|
if (maxY < y) maxY = y;
|
|
}
|
|
}
|
|
}
|
|
|
|
let croppedWidth = maxX - minX;
|
|
let croppedHeight = maxY - minY;
|
|
if (croppedWidth < 0 || croppedHeight < 0) return null;
|
|
let cuttedImageData = originalCtx.getImageData(
|
|
minX,
|
|
minY,
|
|
croppedWidth,
|
|
croppedHeight
|
|
);
|
|
|
|
let croppedCanvas = document.createElement("canvas"),
|
|
croppedCtx = croppedCanvas.getContext("2d");
|
|
|
|
croppedCanvas.width = croppedWidth;
|
|
croppedCanvas.height = croppedHeight;
|
|
croppedCtx.putImageData(cuttedImageData, 0, 0);
|
|
|
|
return croppedCanvas.toDataURL();
|
|
}
|
|
|
|
function resizeCanvas() {
|
|
var ratio = Math.max(window.devicePixelRatio || 1, 1);
|
|
var additionalFactor = 10;
|
|
|
|
signaturePadCanvas.width =
|
|
signaturePadCanvas.offsetWidth * ratio * additionalFactor;
|
|
signaturePadCanvas.height =
|
|
signaturePadCanvas.offsetHeight * ratio * additionalFactor;
|
|
signaturePadCanvas
|
|
.getContext("2d")
|
|
.scale(ratio * additionalFactor, ratio * additionalFactor);
|
|
|
|
signaturePad.clear();
|
|
}
|
|
|
|
new IntersectionObserver((entries, observer) => {
|
|
if (entries.some((entry) => entry.intersectionRatio > 0)) {
|
|
resizeCanvas();
|
|
}
|
|
}).observe(signaturePadCanvas);
|
|
|
|
new ResizeObserver(resizeCanvas).observe(signaturePadCanvas);
|
|
|
|
function addDraggableFromText() {
|
|
const sigText = document.getElementById("sigText").value;
|
|
const font = document.querySelector("select[name=font]").value;
|
|
const fontSize = 100;
|
|
|
|
const canvas = document.createElement("canvas");
|
|
const ctx = canvas.getContext("2d");
|
|
ctx.font = `${fontSize}px ${font}`;
|
|
const textWidth = ctx.measureText(sigText).width;
|
|
const textHeight = fontSize;
|
|
|
|
let paragraphs = sigText.split(/\r?\n/);
|
|
|
|
canvas.width = textWidth;
|
|
canvas.height = paragraphs.length * textHeight * 1.35; // for tails
|
|
ctx.font = `${fontSize}px ${font}`;
|
|
|
|
ctx.textBaseline = "top";
|
|
|
|
let y = 0;
|
|
|
|
paragraphs.forEach((paragraph) => {
|
|
ctx.fillText(paragraph, 0, y);
|
|
y += fontSize;
|
|
});
|
|
|
|
const dataURL = canvas.toDataURL();
|
|
DraggableUtils.createDraggableCanvasFromUrl(dataURL);
|
|
}
|
|
|
|
async function goToFirstOrLastPage(page) {
|
|
if (page) {
|
|
const lastPage = DraggableUtils.pdfDoc.numPages;
|
|
await DraggableUtils.goToPage(lastPage - 1);
|
|
} else {
|
|
await DraggableUtils.goToPage(0);
|
|
}
|
|
}
|
|
|
|
document.getElementById("download-pdf").addEventListener("click", async () => {
|
|
const downloadButton = document.getElementById("download-pdf");
|
|
const originalContent = downloadButton.innerHTML;
|
|
|
|
downloadButton.disabled = true;
|
|
downloadButton.innerHTML = `
|
|
<span class="spinner-border spinner-border-sm" role="status" aria-hidden="true"></span>
|
|
`;
|
|
|
|
try {
|
|
const modifiedPdf = await DraggableUtils.getOverlayedPdfDocument();
|
|
const modifiedPdfBytes = await modifiedPdf.save();
|
|
const blob = new Blob([modifiedPdfBytes], { type: "application/pdf" });
|
|
const link = document.createElement("a");
|
|
link.href = URL.createObjectURL(blob);
|
|
link.download = originalFileName + "_signed.pdf";
|
|
link.click();
|
|
} catch (error) {
|
|
console.error("Error downloading PDF:", error);
|
|
} finally {
|
|
downloadButton.disabled = false;
|
|
downloadButton.innerHTML = originalContent;
|
|
}
|
|
});
|