mirror of
https://github.com/Stirling-Tools/Stirling-PDF.git
synced 2025-06-23 16:05:09 +00:00
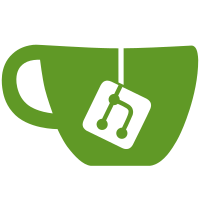
# Description of Changes - PDF split by size to check size of PDF as it splits, avoids issue were a PDFs size is different viewed vs saved due to compression caused by repeated data etc. - Additionally memory enhancements for PDF load to dynamically load in memory vs scratch - PDF Decompress API for PDF testing ## Checklist ### General - [ ] I have read the [Contribution Guidelines](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/CONTRIBUTING.md) - [ ] I have read the [Stirling-PDF Developer Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md) (if applicable) - [ ] I have read the [How to add new languages to Stirling-PDF](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md) (if applicable) - [ ] I have performed a self-review of my own code - [ ] My changes generate no new warnings ### Documentation - [ ] I have updated relevant docs on [Stirling-PDF's doc repo](https://github.com/Stirling-Tools/Stirling-Tools.github.io/blob/main/docs/) (if functionality has heavily changed) - [ ] I have read the section [Add New Translation Tags](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/HowToAddNewLanguage.md#add-new-translation-tags) (for new translation tags only) ### UI Changes (if applicable) - [ ] Screenshots or videos demonstrating the UI changes are attached (e.g., as comments or direct attachments in the PR) ### Testing (if applicable) - [ ] I have tested my changes locally. Refer to the [Testing Guide](https://github.com/Stirling-Tools/Stirling-PDF/blob/main/DeveloperGuide.md#6-testing) for more details.
98 lines
4.2 KiB
Java
98 lines
4.2 KiB
Java
package stirling.software.SPDF.controller.api;
|
|
|
|
import java.awt.geom.AffineTransform;
|
|
import java.io.ByteArrayOutputStream;
|
|
import java.io.IOException;
|
|
|
|
import org.apache.pdfbox.multipdf.LayerUtility;
|
|
import org.apache.pdfbox.pdmodel.PDDocument;
|
|
import org.apache.pdfbox.pdmodel.PDPage;
|
|
import org.apache.pdfbox.pdmodel.PDPageContentStream;
|
|
import org.apache.pdfbox.pdmodel.common.PDRectangle;
|
|
import org.apache.pdfbox.pdmodel.graphics.form.PDFormXObject;
|
|
import org.springframework.beans.factory.annotation.Autowired;
|
|
import org.springframework.http.ResponseEntity;
|
|
import org.springframework.web.bind.annotation.ModelAttribute;
|
|
import org.springframework.web.bind.annotation.PostMapping;
|
|
import org.springframework.web.bind.annotation.RequestMapping;
|
|
import org.springframework.web.bind.annotation.RestController;
|
|
|
|
import io.swagger.v3.oas.annotations.Operation;
|
|
import io.swagger.v3.oas.annotations.tags.Tag;
|
|
|
|
import stirling.software.SPDF.model.api.PDFFile;
|
|
import stirling.software.SPDF.service.CustomPDDocumentFactory;
|
|
import stirling.software.SPDF.utils.WebResponseUtils;
|
|
|
|
@RestController
|
|
@RequestMapping("/api/v1/general")
|
|
@Tag(name = "General", description = "General APIs")
|
|
public class ToSinglePageController {
|
|
|
|
private final CustomPDDocumentFactory pdfDocumentFactory;
|
|
|
|
@Autowired
|
|
public ToSinglePageController(CustomPDDocumentFactory pdfDocumentFactory) {
|
|
this.pdfDocumentFactory = pdfDocumentFactory;
|
|
}
|
|
|
|
@PostMapping(consumes = "multipart/form-data", value = "/pdf-to-single-page")
|
|
@Operation(
|
|
summary = "Convert a multi-page PDF into a single long page PDF",
|
|
description =
|
|
"This endpoint converts a multi-page PDF document into a single paged PDF document. The width of the single page will be same as the input's width, but the height will be the sum of all the pages' heights. Input:PDF Output:PDF Type:SISO")
|
|
public ResponseEntity<byte[]> pdfToSinglePage(@ModelAttribute PDFFile request)
|
|
throws IOException {
|
|
|
|
// Load the source document
|
|
PDDocument sourceDocument = pdfDocumentFactory.load(request.getFileInput().getBytes());
|
|
|
|
// Calculate total height and max width
|
|
float totalHeight = 0;
|
|
float maxWidth = 0;
|
|
for (PDPage page : sourceDocument.getPages()) {
|
|
PDRectangle pageSize = page.getMediaBox();
|
|
totalHeight += pageSize.getHeight();
|
|
maxWidth = Math.max(maxWidth, pageSize.getWidth());
|
|
}
|
|
|
|
// Create new document and page with calculated dimensions
|
|
PDDocument newDocument =
|
|
pdfDocumentFactory.createNewDocumentBasedOnOldDocument(sourceDocument);
|
|
PDPage newPage = new PDPage(new PDRectangle(maxWidth, totalHeight));
|
|
newDocument.addPage(newPage);
|
|
|
|
// Initialize the content stream of the new page
|
|
PDPageContentStream contentStream = new PDPageContentStream(newDocument, newPage);
|
|
contentStream.close();
|
|
|
|
LayerUtility layerUtility = new LayerUtility(newDocument);
|
|
float yOffset = totalHeight;
|
|
|
|
// For each page, copy its content to the new page at the correct offset
|
|
for (PDPage page : sourceDocument.getPages()) {
|
|
PDFormXObject form =
|
|
layerUtility.importPageAsForm(
|
|
sourceDocument, sourceDocument.getPages().indexOf(page));
|
|
AffineTransform af =
|
|
AffineTransform.getTranslateInstance(
|
|
0, yOffset - page.getMediaBox().getHeight());
|
|
layerUtility.wrapInSaveRestore(newPage);
|
|
String defaultLayerName = "Layer" + sourceDocument.getPages().indexOf(page);
|
|
layerUtility.appendFormAsLayer(newPage, form, af, defaultLayerName);
|
|
yOffset -= page.getMediaBox().getHeight();
|
|
}
|
|
|
|
ByteArrayOutputStream baos = new ByteArrayOutputStream();
|
|
newDocument.save(baos);
|
|
newDocument.close();
|
|
sourceDocument.close();
|
|
|
|
byte[] result = baos.toByteArray();
|
|
return WebResponseUtils.bytesToWebResponse(
|
|
result,
|
|
request.getFileInput().getOriginalFilename().replaceFirst("[.][^.]+$", "")
|
|
+ "_singlePage.pdf");
|
|
}
|
|
}
|