mirror of
https://code.castopod.org/adaures/castopod
synced 2025-04-23 01:01:20 +00:00
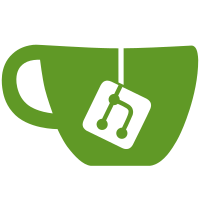
- create permalink-edit web component with slug editing and permalink copy functionalities - add @github/clipboard-copy-element - update npm packages - replace vscode extension lit-html with lit-plugin to get css intellisense
38 lines
1.3 KiB
TypeScript
38 lines
1.3 KiB
TypeScript
// Original code from: https://gist.github.com/hagemann/382adfc57adbd5af078dc93feef01fe1
|
|
const slugify = (text: string) => {
|
|
const a =
|
|
"àáâäæãåāăąçćčđďèéêëēėęěğǵḧîïíīįìłḿñńǹňôöòóœøōõőṕŕřßśšşșťțûüùúūǘůűųẃẍÿýžźż·/_,:;";
|
|
const b =
|
|
"aaaaaaaaaacccddeeeeeeeegghiiiiiilmnnnnoooooooooprrsssssttuuuuuuuuuwxyyzzz------";
|
|
const p = new RegExp(a.split("").join("|"), "g");
|
|
|
|
return text
|
|
.toString()
|
|
.toLowerCase()
|
|
.replace(/\s+/g, "-") // Replace spaces with -
|
|
.replace(p, (c) => b.charAt(a.indexOf(c))) // Replace special characters
|
|
.replace(/&/g, "-and-") // Replace & with 'and'
|
|
.replace(/[^\w-]+/g, "") // Remove all non-word characters
|
|
.replace(/--+/g, "-") // Replace multiple - with single -
|
|
.replace(/^-+/, "") // Trim - from start of text
|
|
.replace(/-+$/, ""); // Trim - from end of text
|
|
};
|
|
|
|
const Slugify = (): void => {
|
|
const title: HTMLInputElement | null = document.querySelector(
|
|
"input[data-slugify='title']"
|
|
);
|
|
const slug: HTMLInputElement | null = document.querySelector(
|
|
"input[data-slugify='slug']"
|
|
);
|
|
|
|
if (title && slug) {
|
|
title.addEventListener("input", () => {
|
|
slug.value = slugify(title.value);
|
|
slug.dispatchEvent(new Event("change"));
|
|
});
|
|
}
|
|
};
|
|
|
|
export default Slugify;
|