mirror of
https://code.castopod.org/adaures/castopod
synced 2025-05-10 16:25:47 +00:00
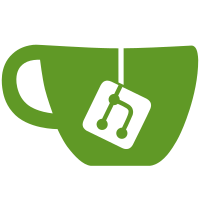
- resize uploaded image to thumbnail, medium, large, feed, and id3 formats - set image url formats where adapted in views - set format sizes and extensions in Images config file for customization - add validation for image uploads: `min_dims` and `is_image_squared` - update codeigniter4 and myth-auth php packages to latest develop versions - update npm packages to latest versions - update public/.htaccess closes #6
77 lines
2.0 KiB
PHP
77 lines
2.0 KiB
PHP
<?php
|
|
|
|
/**
|
|
* @copyright 2020 Podlibre
|
|
* @license https://www.gnu.org/licenses/agpl-3.0.en.html AGPL3
|
|
* @link https://castopod.org/
|
|
*/
|
|
|
|
/**
|
|
* Saves a file to the corresponding podcast folder in `public/media`
|
|
*
|
|
* @param \CodeIgniter\HTTP\Files\UploadedFile|\CodeIgniter\Files\File $file
|
|
* @param string $podcast_name
|
|
* @param string $file_name
|
|
*
|
|
* @return string The episode's file path in media root
|
|
*/
|
|
function save_podcast_media($file, $podcast_name, $media_name)
|
|
{
|
|
$file_name = $media_name . '.' . $file->getExtension();
|
|
|
|
$mediaRoot = config('App')->mediaRoot;
|
|
|
|
if (!file_exists($mediaRoot . '/' . $podcast_name)) {
|
|
mkdir($mediaRoot . '/' . $podcast_name, 0777, true);
|
|
touch($mediaRoot . '/' . $podcast_name . '/index.html');
|
|
}
|
|
|
|
// move to media folder and overwrite file if already existing
|
|
$file->move($mediaRoot . '/' . $podcast_name . '/', $file_name, true);
|
|
|
|
return $podcast_name . '/' . $file_name;
|
|
}
|
|
|
|
function download_file($fileUrl)
|
|
{
|
|
$tmpFilename =
|
|
time() .
|
|
'_' .
|
|
bin2hex(random_bytes(10)) .
|
|
'.' .
|
|
pathinfo($fileUrl, PATHINFO_EXTENSION);
|
|
$tmpFilePath = WRITEPATH . 'uploads/' . $tmpFilename;
|
|
file_put_contents($tmpFilePath, file_get_contents($fileUrl));
|
|
|
|
return new \CodeIgniter\Files\File($tmpFilePath);
|
|
}
|
|
|
|
/**
|
|
* Prefixes the root media path to a given uri
|
|
*
|
|
* @param mixed $uri URI string or array of URI segments
|
|
* @return string
|
|
*/
|
|
function media_path($uri = ''): string
|
|
{
|
|
// convert segment array to string
|
|
if (is_array($uri)) {
|
|
$uri = implode('/', $uri);
|
|
}
|
|
$uri = trim($uri, '/');
|
|
|
|
return config('App')->mediaRoot . '/' . $uri;
|
|
}
|
|
|
|
/**
|
|
* Return the media base URL to use in views
|
|
*
|
|
* @param mixed $uri URI string or array of URI segments
|
|
* @param string $protocol
|
|
* @return string
|
|
*/
|
|
function media_url($uri = '', string $protocol = null): string
|
|
{
|
|
return base_url(config('App')->mediaRoot . '/' . $uri, $protocol);
|
|
}
|