mirror of
https://code.castopod.org/adaures/castopod
synced 2025-04-23 01:01:20 +00:00
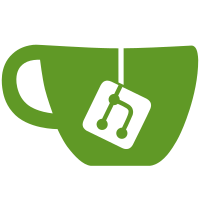
- update myth-auth and codeigniter to latest develop changes - improve permission check: remove all dynamic permissions per podcast and overwrite myth-auth services and permission filter - remove unnecessary code because of myth-auth upgrade - refactor some controller code for better clarity - add remaining seeders in docs closes #19, #20
116 lines
3.7 KiB
PHP
116 lines
3.7 KiB
PHP
<?php namespace App\Filters;
|
|
|
|
use App\Models\PodcastModel;
|
|
use Config\Services;
|
|
use CodeIgniter\HTTP\RequestInterface;
|
|
use CodeIgniter\HTTP\ResponseInterface;
|
|
use CodeIgniter\Filters\FilterInterface;
|
|
use Myth\Auth\Exceptions\PermissionException;
|
|
|
|
class Permission implements FilterInterface
|
|
{
|
|
/**
|
|
* Do whatever processing this filter needs to do.
|
|
* By default it should not return anything during
|
|
* normal execution. However, when an abnormal state
|
|
* is found, it should return an instance of
|
|
* CodeIgniter\HTTP\Response. If it does, script
|
|
* execution will end and that Response will be
|
|
* sent back to the client, allowing for error pages,
|
|
* redirects, etc.
|
|
*
|
|
* @param \CodeIgniter\HTTP\RequestInterface $request
|
|
* @param array|null $params
|
|
*
|
|
* @return mixed
|
|
*/
|
|
public function before(RequestInterface $request, $params = null)
|
|
{
|
|
if (!function_exists('logged_in')) {
|
|
helper('auth');
|
|
}
|
|
|
|
if (empty($params)) {
|
|
return;
|
|
}
|
|
|
|
$authenticate = Services::authentication();
|
|
|
|
// if no user is logged in then send to the login form
|
|
if (!$authenticate->check()) {
|
|
session()->set('redirect_url', current_url());
|
|
return redirect('login');
|
|
}
|
|
|
|
helper('misc');
|
|
$authorize = Services::authorization();
|
|
$router = Services::router();
|
|
$routerParams = $router->params();
|
|
$result = false;
|
|
|
|
// Check if user has at least one of the permissions
|
|
foreach ($params as $permission) {
|
|
// check if permission is for a specific podcast
|
|
if (
|
|
(startsWith($permission, 'podcast-') ||
|
|
startsWith($permission, 'podcast_episodes-')) &&
|
|
count($routerParams) > 0
|
|
) {
|
|
if (
|
|
$group_id = (new PodcastModel())->getContributorGroupId(
|
|
$authenticate->id(),
|
|
$routerParams[0]
|
|
)
|
|
) {
|
|
if (
|
|
$authorize->groupHasPermission($permission, $group_id)
|
|
) {
|
|
$result = true;
|
|
break;
|
|
}
|
|
}
|
|
} elseif (
|
|
$authorize->hasPermission($permission, $authenticate->id())
|
|
) {
|
|
$result = true;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (!$result) {
|
|
if ($authenticate->silent()) {
|
|
$redirectURL = session('redirect_url') ?? '/';
|
|
unset($_SESSION['redirect_url']);
|
|
return redirect()
|
|
->to($redirectURL)
|
|
->with('error', lang('Auth.notEnoughPrivilege'));
|
|
} else {
|
|
throw new PermissionException(lang('Auth.notEnoughPrivilege'));
|
|
}
|
|
}
|
|
}
|
|
|
|
//--------------------------------------------------------------------
|
|
|
|
/**
|
|
* Allows After filters to inspect and modify the response
|
|
* object as needed. This method does not allow any way
|
|
* to stop execution of other after filters, short of
|
|
* throwing an Exception or Error.
|
|
*
|
|
* @param \CodeIgniter\HTTP\RequestInterface $request
|
|
* @param \CodeIgniter\HTTP\ResponseInterface $response
|
|
* @param array|null $arguments
|
|
*
|
|
* @return void
|
|
*/
|
|
public function after(
|
|
RequestInterface $request,
|
|
ResponseInterface $response,
|
|
$arguments = null
|
|
) {
|
|
}
|
|
|
|
//--------------------------------------------------------------------
|
|
}
|