mirror of
https://code.castopod.org/adaures/castopod
synced 2025-06-23 16:05:34 +00:00
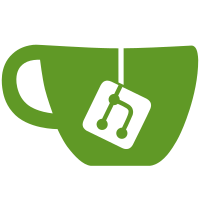
- add .editorconfig file - format all files to comply with castopod's coding style - switch parsedown dependency with commonmark library to better follow commonmark spec for markdown - add prettier command to format all project files at once closes #16
66 lines
1.7 KiB
PHP
66 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace App\Authorization;
|
|
|
|
class PermissionModel extends \Myth\Auth\Authorization\PermissionModel
|
|
{
|
|
/**
|
|
* Checks to see if a user, or one of their groups,
|
|
* has a specific permission.
|
|
*
|
|
* @param $userId
|
|
* @param $permissionId
|
|
*
|
|
* @return bool
|
|
*/
|
|
public function doesGroupHavePermission(
|
|
int $groupId,
|
|
int $permissionId
|
|
): bool {
|
|
// Check group permissions and take advantage of caching
|
|
$groupPerms = $this->getPermissionsForGroup($groupId);
|
|
|
|
return count($groupPerms) &&
|
|
array_key_exists($permissionId, $groupPerms);
|
|
}
|
|
|
|
/**
|
|
* Gets all permissions for a group in a way that can be
|
|
* easily used to check against:
|
|
*
|
|
* [
|
|
* id => name,
|
|
* id => name
|
|
* ]
|
|
*
|
|
* @param int $groupId
|
|
*
|
|
* @return array
|
|
*/
|
|
public function getPermissionsForGroup(int $groupId): array
|
|
{
|
|
if (!($found = cache("group{$groupId}_permissions"))) {
|
|
$groupPermissions = $this->db
|
|
->table('auth_groups_permissions')
|
|
->select('id, auth_permissions.name')
|
|
->join(
|
|
'auth_permissions',
|
|
'auth_permissions.id = permission_id',
|
|
'inner'
|
|
)
|
|
->where('group_id', $groupId)
|
|
->get()
|
|
->getResultObject();
|
|
|
|
$found = [];
|
|
foreach ($groupPermissions as $row) {
|
|
$found[$row->id] = strtolower($row->name);
|
|
}
|
|
|
|
cache()->save("group{$groupId}_permissions", $found, 300);
|
|
}
|
|
|
|
return $found;
|
|
}
|
|
}
|