mirror of
https://github.com/DocNR/POWR.git
synced 2025-04-23 01:01:27 +00:00
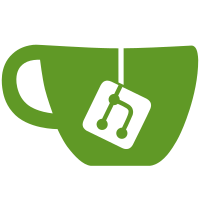
* Add style prop to UserAvatar component for better customization * Refactor UserAvatar to use getAvatarSeed utility for consistent avatar generation * Fix React hook ordering issues in profile/overview.tsx to prevent crashes during auth state changes * Add proper state initialization and cleanup during authentication transitions * Ensure consistent fallback avatar display for unauthenticated users These changes improve stability during login/logout operations and provide better visual continuity with Robohash avatars when profile images aren't available.
5.1 KiB
5.1 KiB
ARCHIVED DOCUMENT: This document is outdated and kept for historical reference only. Please refer to Migration Guide for up-to-date information.
Workout History API Migration Guide
Overview
We've consolidated our workout history services and hooks to provide a more consistent and maintainable API. This guide will help you migrate from the old APIs to the new unified API.
Why We're Consolidating
The previous implementation had several overlapping services:
- WorkoutHistoryService - Basic service for local database operations
- EnhancedWorkoutHistoryService - Extended service with advanced features
- NostrWorkoutHistoryService - Service for Nostr integration
- useNostrWorkoutHistory Hook - Hook for fetching workouts from Nostr
This led to:
- Duplicate code and functionality
- Inconsistent APIs
- Confusion about which service to use for what purpose
- Difficulty maintaining and extending the codebase
New Architecture
The new architecture consists of:
- UnifiedWorkoutHistoryService - A single service that combines all functionality
- useWorkoutHistory - A single hook that provides access to all workout history features
Service Migration
Before:
// Using WorkoutHistoryService
const workoutHistoryService = new WorkoutHistoryService(db);
const localWorkouts = await workoutHistoryService.getAllWorkouts();
// Using NostrWorkoutHistoryService
const nostrWorkoutHistoryService = new NostrWorkoutHistoryService(db);
const allWorkouts = await nostrWorkoutHistoryService.getAllWorkouts({
includeNostr: true,
isAuthenticated: true
});
After:
// Using unified UnifiedWorkoutHistoryService
const workoutHistoryService = new UnifiedWorkoutHistoryService(db);
// For local workouts only
const localWorkouts = await workoutHistoryService.getAllWorkouts({
includeNostr: false
});
// For all workouts (local + Nostr)
const allWorkouts = await workoutHistoryService.getAllWorkouts({
includeNostr: true,
isAuthenticated: true
});
Hook Migration
Before:
// Using useNostrWorkoutHistory
const { workouts, loading } = useNostrWorkoutHistory();
// Or manually creating a service in a component
const db = useSQLiteContext();
const workoutHistoryService = React.useMemo(() => new WorkoutHistoryService(db), [db]);
// Then loading workouts
const loadWorkouts = async () => {
const workouts = await workoutHistoryService.getAllWorkouts();
setWorkouts(workouts);
};
After:
// Using unified useWorkoutHistory
const {
workouts,
loading,
refresh,
getWorkoutsByDate,
publishWorkoutToNostr
} = useWorkoutHistory({
includeNostr: true,
realtime: true,
filters: { type: ['strength'] } // Optional filters
});
Key Benefits of the New API
- Simplified Interface: One service and one hook to learn and use
- Real-time Updates: Built-in support for real-time Nostr updates
- Consistent Filtering: Unified filtering across local and Nostr workouts
- Better Type Safety: Improved TypeScript types and interfaces
- Reduced Boilerplate: Less code needed in components
Examples of Updated Components
Calendar View
// Before
const workoutHistoryService = React.useMemo(() => new WorkoutHistoryService(db), [db]);
const loadWorkouts = async () => {
const allWorkouts = await workoutHistoryService.getAllWorkouts();
setWorkouts(allWorkouts);
};
// After
const {
workouts: allWorkouts,
loading,
refresh,
getWorkoutsByDate
} = useWorkoutHistory({
includeNostr: true,
realtime: true
});
Workout History Screen
// Before
const workoutHistoryService = React.useMemo(() => new WorkoutHistoryService(db), [db]);
const loadWorkouts = async () => {
let allWorkouts = [];
if (includeNostr) {
allWorkouts = await workoutHistoryService.getAllWorkouts();
} else {
allWorkouts = await workoutHistoryService.filterWorkouts({
source: ['local']
});
}
setWorkouts(allWorkouts);
};
// After
const {
workouts: allWorkouts,
loading,
refresh
} = useWorkoutHistory({
includeNostr,
filters: includeNostr ? undefined : { source: ['local'] },
realtime: true
});
Workout Detail Screen
// Before
const workoutHistoryService = React.useMemo(() => new WorkoutHistoryService(db), [db]);
const loadWorkout = async () => {
const workoutDetails = await workoutHistoryService.getWorkoutDetails(id);
setWorkout(workoutDetails);
};
// After
const { getWorkoutDetails, publishWorkoutToNostr } = useWorkoutHistory();
const loadWorkout = async () => {
const workoutDetails = await getWorkoutDetails(id);
setWorkout(workoutDetails);
};
Timeline
- The old APIs are now deprecated and will be removed in a future release
- Please migrate to the new APIs as soon as possible
- If you encounter any issues during migration, please contact the development team