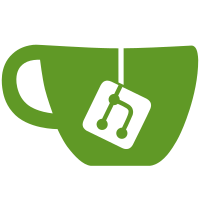
* Add style prop to UserAvatar component for better customization * Refactor UserAvatar to use getAvatarSeed utility for consistent avatar generation * Fix React hook ordering issues in profile/overview.tsx to prevent crashes during auth state changes * Add proper state initialization and cleanup during authentication transitions * Ensure consistent fallback avatar display for unauthenticated users These changes improve stability during login/logout operations and provide better visual continuity with Robohash avatars when profile images aren't available.
3.9 KiB
Profile Section Documentation
Last Updated: 2025-04-02
Status: Implemented
Related To: Nostr Integration, Analytics
Overview
The Profile section provides users with a comprehensive interface for managing their personal information, viewing activity, tracking progress, and configuring application settings. This documentation covers all aspects of the Profile tab implementation, from high-level architecture to specific implementation details.
Documentation Structure
Core Documentation
- Profile Tab Overview - High-level overview of the Profile tab structure and architecture
- Authentication Patterns - Technical details on authentication implementation and React hook ordering
Tab Implementation Details
- Profile (Overview) Tab - User profile display and social feed
- Activity Tab - User activity summary and recent workouts
- Progress Tab - Workout analytics and progress tracking
- Settings Tab - Application and account settings
Technical Features
- Progress Tracking - Implementation details for workout progress tracking
- Follower Statistics - NostrBand integration for follower statistics
Key Technical Concepts
The Profile section implements several key technical concepts that are worth highlighting:
Authentication Pattern
The Profile tab implements a consistent authentication pattern that ensures React hook ordering is maintained while supporting conditional rendering based on authentication state. See Authentication Patterns for details.
Components and Data Flow
graph TD
A[Profile Tab Navigator] --> B[Overview Tab]
A --> C[Activity Tab]
A --> D[Progress Tab]
A --> E[Settings Tab]
B --> F[Profile Header]
B --> G[Social Feed]
B --> H[Follower Stats]
C --> I[Workout Stats]
C --> J[Personal Records]
C --> K[Recent Workouts]
D --> L[Analytics Charts]
D --> M[Period Filter]
D --> N[Nostr Toggle]
E --> O[Account Settings]
E --> P[App Settings]
E --> Q[Logout]
Hook Management
The Profile section carefully manages React hooks to ensure they are called consistently regardless of conditional rendering:
// Always define hooks at the top level, regardless of authentication state
const { isAuthenticated } = useNDKCurrentUser();
const someHook = useSomeHook();
// Only after all hooks are defined, use conditional rendering
if (!isAuthenticated) {
return <LoginComponent />;
}
// Continue with authenticated UI
Nostr Integration
The Profile section deeply integrates with Nostr for user profiles, follower statistics, and social feed:
// Example of Nostr integration in the profile
const { currentUser, isAuthenticated } = useNDKCurrentUser();
const { followersCount, followingCount } = useProfileStats({
pubkey: currentUser?.pubkey || ''
});
Implementation Challenges
Several key implementation challenges were addressed in the Profile section:
- React Hook Consistency: Ensuring React hooks are called in the same order regardless of authentication state
- Feed Data Transformation: Transforming feed data from different sources into a consistent format
- Analytics Visualization: Creating user-friendly visualizations of workout analytics
- Offline Support: Graceful handling of offline state throughout the Profile section
Related Documentation
- Nostr Integration - General Nostr integration documentation
- Analytics Implementation - Implementation details for analytics
- Theme System - App theming system